Create a login form
Following the previous lesson, we will create a login form.
In other words, we will also create a login feature. Let's create a login feature.
The login form is not so different from the registration form. By design.
There is an ID field, a password field, a login button, and a sign up button below. If you press the sign up button, you will be directed to the sign up page.
/htdocs/myProject/member/signInForm.php
<?php include "../include/session.php"; // If you are already logged in if(isset($_SESSION['ses_userid'])){ echo "<script>alert('The wrong approach.');location.href='/myProject/'</script>"; exit; } ?> <!doctype html> <html> <head> <meta charset="UTF-8" /> <title>coreasur</title> <script type="text/javascript" src="http://code.jquery.com/jquery-3.4.1.min.js" ></script> <script type="text/javascript" src="/myProject/js/mySignInForm.js"></script> <link rel="stylesheet" href="/myProject/css/mySignInForm.css" /> </head> <body> <div id="wrap"> <div id="container"> <h1 class="title">Welcome</h1> <form name="singIn" action="./signIn.php" method="post" onsubmit="return checkSubmit()"> <div class="line"> <label for="memberId">ID</label> <div class="inputArea"> <input type="text" name="memberId" id="memberId" class="memberId" /> </div> </div> <div class="line"> <label for="memberPw">password</label> <div class="inputArea"> <input type="password" name="memberPw" id="memberPw" class="memberPw" /> </div> </div> <div class="line"> <input type="submit" value="signIn" class="submit" /> </div> </form> <h1 class="title"><a href="./signUpForm.php">go to signUp</a></h1> </div> </div> </body> </html>
atom
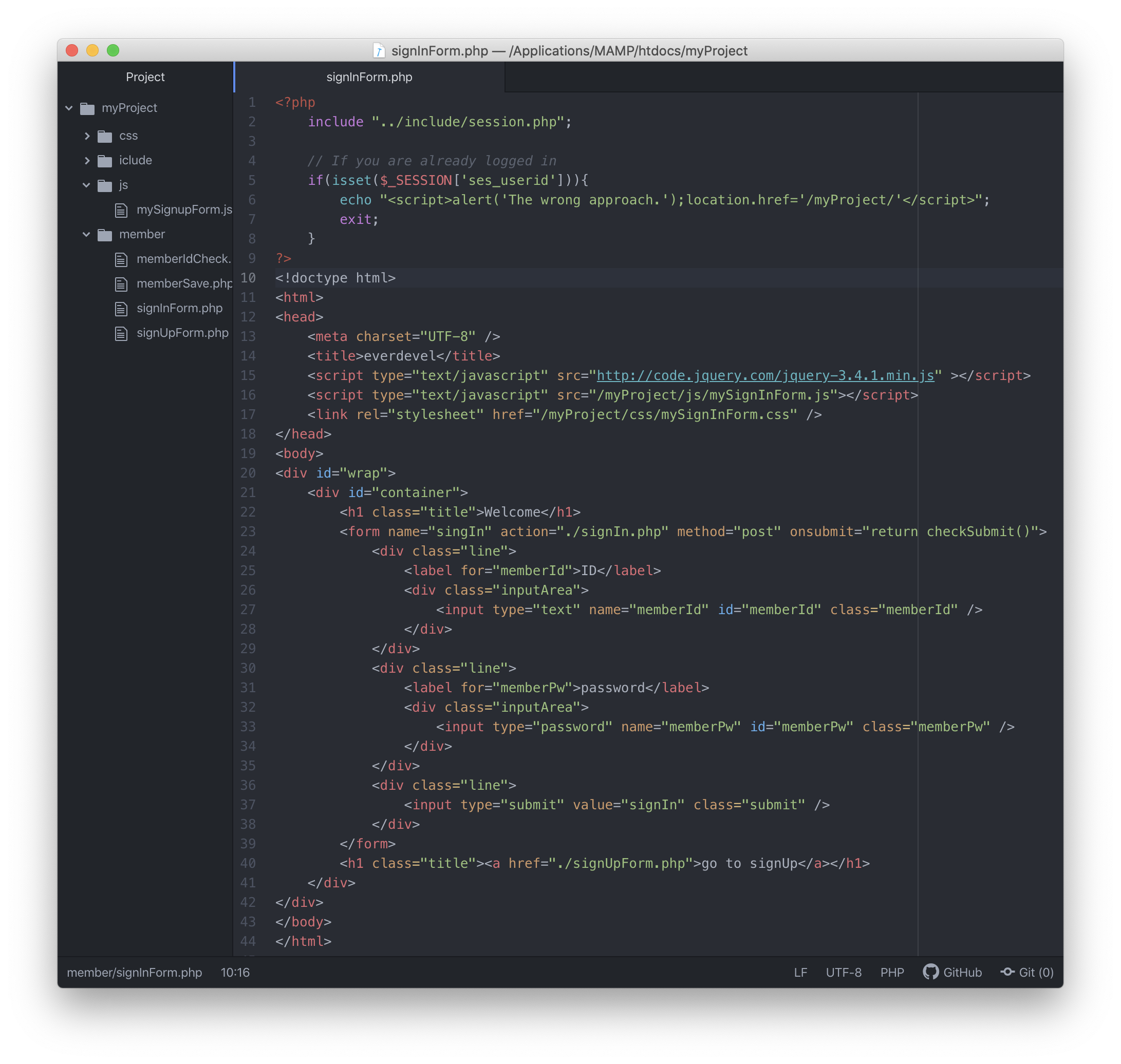
Next is the source to control whether or not to enter the ID and password.
htdocs/myProject/js/mySignInForm.js
function checkSubmit(){ var memberId = $('.memberId'); var memberPw = $('.memberPw'); if(memberId.val() == ''){ alert('input ID.'); return false; } if(memberPw.val() == ''){ alert('input Password.'); return false; } return true; }
atom
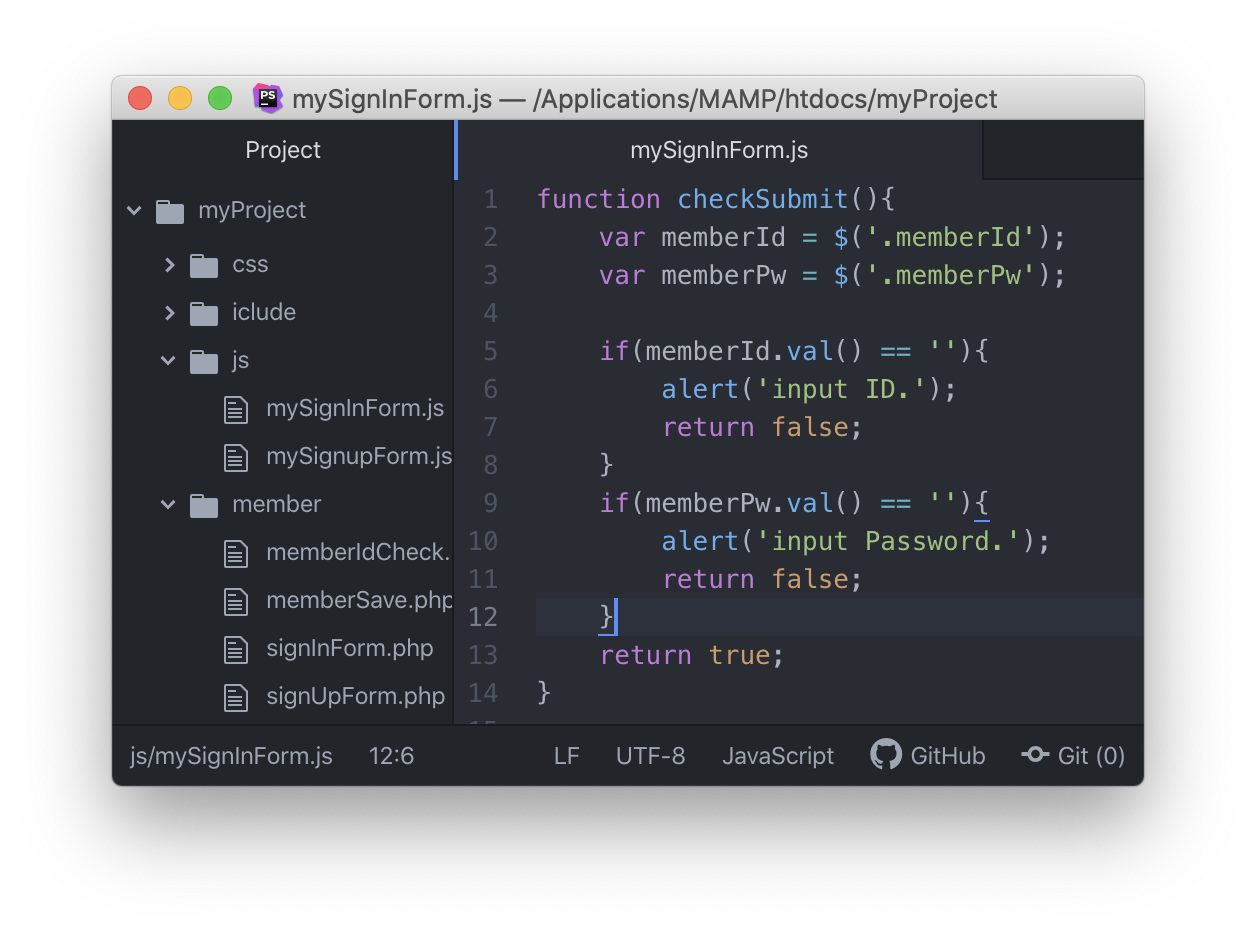
Next, css Source
htdocs/myProject/css/mySignInForm.css
@charset "utf-8"; /* css reset */ div,span,body{margin:0;padding:0} #wrap{clear:both;float:left;width:100%;background:hotpink} #container{clear:both;width:800px;_border:1px solid #fff;margin:3% auto} #container h1{clear:both;float:left;width:100%;text-align:center;color:#fff} #container h1 a{color:#fff;text-decoration:none} .line{clear:both;float:left;width:100%;border:1px solid #fff;margin-bottom:3%;padding:10px 0} .line label{float:left;min-width:100px;margin-left:3%;color:#fff} .line .inputArea{float:left;} .line .inputArea input{float:left;width:370px;height:30px;margin:0px 0 0 0;padding-left:10px} .submit{clear:both;float:left;width:100%;height:50px;background:#fff;border:0;font-size:20px;color:hotpink}
atom
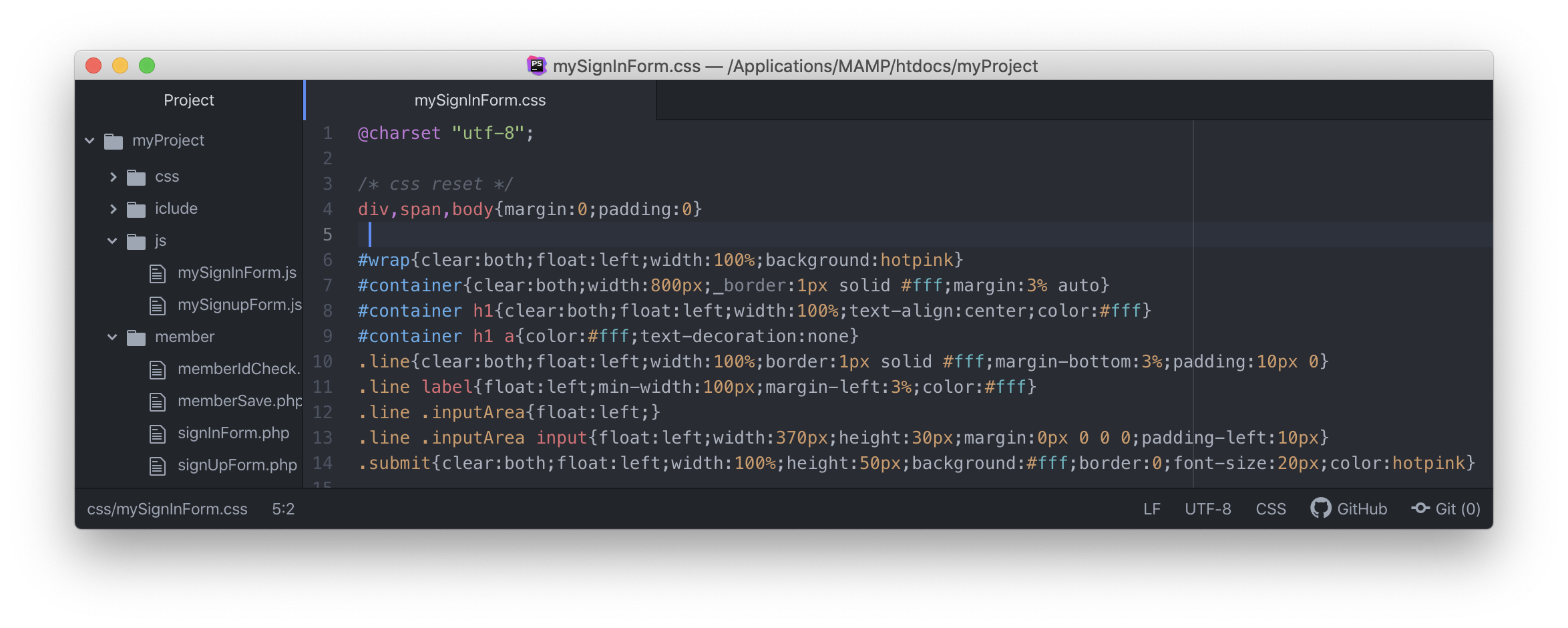
This page itself is so plain that you don't need to explain it. Let's move on to the login process.
Then I'll move on.
run & check signIn page
url : http://localhost/myProject/member/signInForm.php
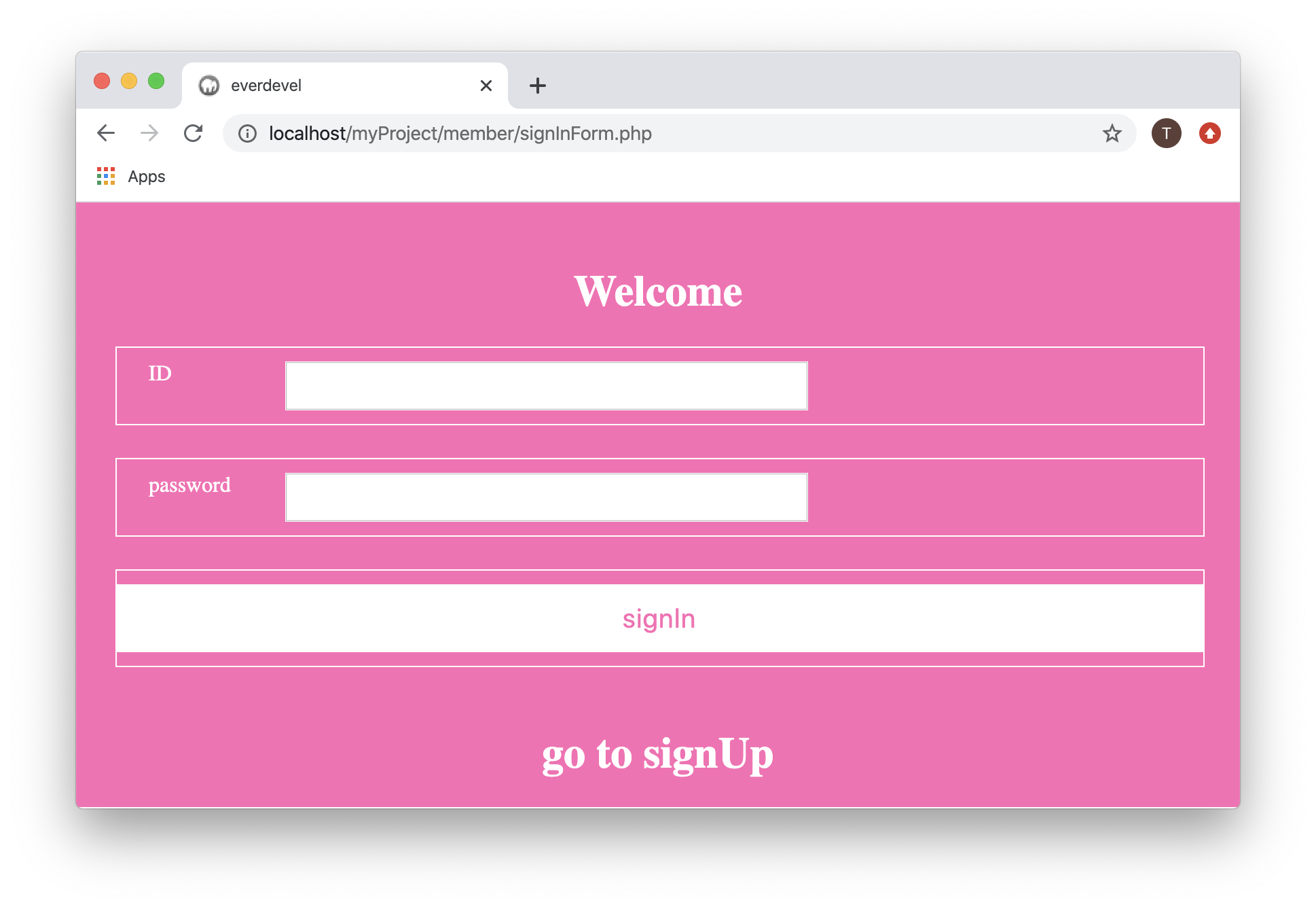