Using the constructor
The constructor is the first method automatically executed when instantiating a class.
In other words, the constructor is executed automatically just by creating a class.
How To Create Constructor
function __construct(){}
So let's do it. Let's use the Car class we used earlier.
Do I have to start it up before the car can move?
<?php class Car { public $wheels; public $doors = 4; protected $color = 4; private $size; private $company; function __construct() { echo "I started to run my car."; } public function run() { return "The car is running."; } protected function stop() { return "car stops."; } protected function turn() { return "turnning car"; } } $honda = new Car; ?>
Result of the code above
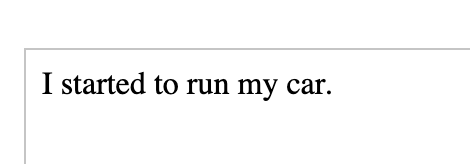
In the code above, I didn't call any methods, I just created an instance.
When you create an instance, the constructor works automatically.
There is also a destructor.
Next time, let's look at the destructor. ^-^ *