Passing data to the constructor
You learned about constructors earlier.
Let's see how to pass data to the constructor.
It's no different from using a parameter when declaring a function and using arguments when calling a function. ^^
How to pass data to the constructor
variable = new class name(argument, argument2, argument3)
How to receive data from the constructor
function __construct(parameter, parameter2, parameter3){}
So let's do it. Let's use the Car class we used earlier.
<?php class Car { public $wheels; public $doors = 4; protected $color = 4; private $size; private $company; function __construct($company) { echo "I started to run my car {$company}."; } public function run() { return "The car is running."; } protected function stop() { return "car stops."; } protected function turn() { return "turnning car"; } } $mercedes = new Car('made by benz'); ?>
Result of the code above
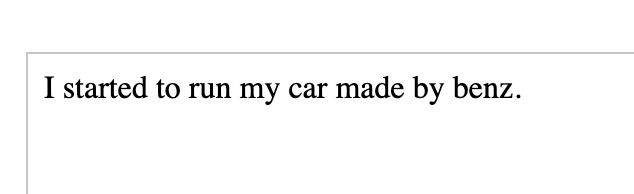
passing two, three, or four data to the constructor is no different from that of a function.
<?php class Car { public $wheels; public $doors = 4; protected $color = 4; private $size; private $company; function __construct($company, $color, $machine) { echo "Start up to operate a {$color} {$machine} made by {$company}"; } public function run() { return "The car is running."; } protected function stop() { return "car stops."; } protected function turn() { return "turnning car"; } } $mercedes = new Car('benz', 'red', 'motorbike'); ?>
Result
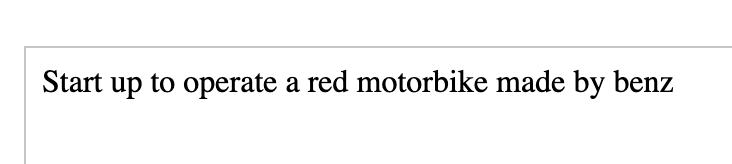